Heads up! This blog is for Laravel/Livewire developers looking to enhance their users’ experience, especially if you’re collecting information through fancy forms designed by the designer or providing a search feature. Once you have received the UI/UX design from your Figma team. It’s now on your to how to keep the website interactive. Have you ever wondered what a multi category or multi-table searchable dropdown is and how it operates? Would you like to enhance the user experience of your Laravel web application by enabling users to perform multi-table/category live searches using Livewire? If so, this article is for you. We’re excited to share a series of articles based on our real-world development and design experiences, aimed at helping you improve your application.
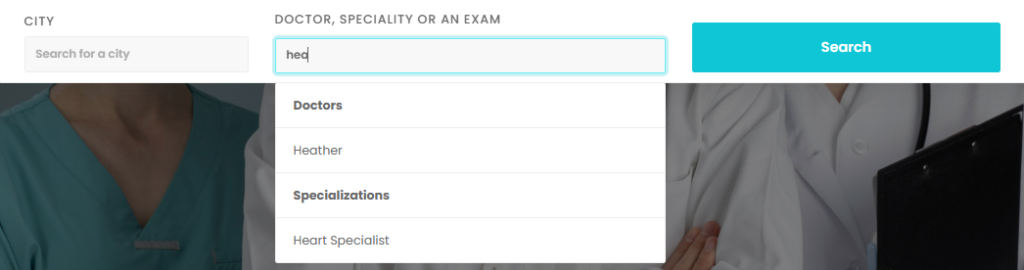
Enhance User Experience by Creating a Dynamic Multi Table Searchable Dropdown with Livewire and Bootstrap
First, we will examine the use case, followed by a discussion of the traditional solutions that our developers replaced with a dynamic multi-table searchable dropdown using Livewire.
Let me share some details about the application our team was developing: a digital healthcare platform aimed at transforming the local healthcare market by connecting doctors with patients. We envisioned a single search feature on the homepage where users could enter a doctor’s name to view a list of relevant doctors. The dropdown would display all doctors matching the input name, as well as related specializations and exams. For example, if a user types “hea,” it would show both “Heather” and “Heart Specialist.” As the search input narrows like typing “Heather”, the results would refine to only display “Heather.” This search functionality would query both the specialization and doctors tables.
Why a Multi Table Searchable Dropdown was Necessary?
In the context of our digital healthcare platform, a multi table searchable dropdown was essential for several key reasons:
- Streamlined User Experience: By consolidating the search functionality into a single dropdown, users could easily access information about doctors, specializations, and exams without needing to navigate multiple fields (multiple dropdowns). This streamlined approach enhances the overall user experience (better UX was our top priority along-side application performance/core functionalities).
- Rapid Information Retrieval: Users can quickly find relevant information by typing just a few letters. For instance, entering “hea” displays both “Heather” and “Heart Specialist” simultaneously. This rapid retrieval is crucial in a healthcare setting where time is often of the essence.
- Dynamic and Intuitive Interaction: Unlike traditional dropdowns that present static lists, a multi-table searchable dropdown offers real-time suggestions based on user input. This dynamic feature makes searching more engaging and efficient, allowing users to see immediate results as they type.
- Reduced Complexity: A single searchable dropdown minimizes the cognitive load on users. They don’t have to remember which category to search in, reducing frustration and improving satisfaction with the platform.
- Simplified Data Management: Integrating multiple tables into one searchable feature allowed us to create a more organized codebase. In traditional methods, developers would need to build and maintain separate dropdowns and handling logic for each category, which can complicate the overall structure.
- Scalability for Future Growth: As the platform evolves and additional categories or data types are introduced, a multi table searchable dropdown can easily adapt to these changes without requiring significant redesign efforts.
- Enhanced User Engagement: By providing a seamless search experience that yields immediate and relevant results, users are more likely to engage with the platform, leading to improved retention and satisfaction rates.
Cut it short, the multi-table searchable dropdown not only addresses the limitations of traditional search methods but also significantly enhances user interaction and data accessibility on our healthcare platform.
Implementing a Multi Table Searchable Dropdown with Livewire
Let’s explore the implementation of a dynamic multi-table searchable dropdown using Livewire in a digital healthcare platform. This feature allows users to search for doctors, specializations, and exams simultaneously, enhancing user experience by providing real-time suggestions as they type. The following code snippets illustrate the Livewire component and view setup, showcasing how to efficiently manage multiple data queries and present the results in a unified interface.
Livewire View Code for Multi Table Searchable Dropdown
<div class="col-md-6 col-lg-4 col-xl-5 pt-6 pt-lg-0 order-2">
<label class="text-uppercase font-weight-500 letter-spacing-093">Doctor, speciality or an exam</label>
<div class="position-relative">
<input type="text" name="selection"
class="form-control"
placeholder="Search for doctors, specialities or exams"
wire:model.defer="genearicSearch"
wire:keyup="searchDocsAndSpecializations">
<ul class="list-group mt-2 position-absolute w-100" style="display: {{ $showDocSpeList ? 'block' : 'none' }}; z-index: 1000;">
@if(count($matchingDoctors) > 0)
<li class="list-group-item font-weight-bold">Doctors</li>
@foreach($matchingDoctors as $doctor)
<li class="list-group-item" style="cursor: pointer;" wire:click="selectDoctor('{{ $doctor['name'] }}')">
{{ $doctor['name'] }}
</li>
@endforeach
@endif
@if(count($matchingSpecializations) > 0)
<li class="list-group-item font-weight-bold">Specializations</li>
@foreach($matchingSpecializations as $specialization)
<li class="list-group-item" style="cursor: pointer;" wire:click="selectSpecialization('{{ $specialization['name'] }}')">
{{ $specialization['name'] }}
</li>
@endforeach
@endif
@if(count($matchingExams) > 0)
<li class="list-group-item font-weight-bold">Exams</li>
@foreach($matchingExams as $exam)
<li class="list-group-item" style="cursor: pointer;" wire:click="selectExam('{{ $exam['exam_name'] }}')">
{{ $exam['exam_name'] }}
</li>
@endforeach
@endif
@if(count($matchingDoctors) === 0 && count($matchingSpecializations) === 0 && count($matchingExams) === 0)
<li class="list-group-item">No results found</li>
@endif
</ul>
</div>
</div>
<input type="text" style="display:none" name="selection_type" id="selection_type" wire:model="selectionType">
Livewire Class Code
class ResourcesSearch extends Component
{
public $matchingDoctors = [], $matchingSpecializations = [], $matchingExams = [];
public $showDocSpeList = false;
public $genearicSearch = '', $searchSelection = '', $selectionType = '';
public function searchDocsAndSpecializations()
{
if ($this->genearicSearch) {
$this->matchingDoctors = Doctor::where('name', 'LIKE', "%{$this->genearicSearch}%")->get();
$this->matchingSpecializations = Specialization::where('name', 'LIKE', "%{$this->genearicSearch}%")->get();
$this->matchingExams = Exam::where('exam_name', 'LIKE', "%{$this->genearicSearch}%")->get();
$this->showDocSpeList = true;
} else {
$this->resetSearchResults();
}
}
public function resetSearchResults() {
$this->matchingDoctors = [];
$this->matchingSpecializations = [];
$this->matchingExams = [];
$this->showDocSpeList = false;
}
public function selectSpecialization($specialization)
{
$this->searchSelection = $specialization;
$this->selectionType = 'specialization';
$this->showDocSpeList = false;
}
public function selectExam($exam)
{
$this->searchSelection = $exam;
$this->selectionType = 'exam';
$this->showDocSpeList = false;
}
public function selectDoctor($doctor)
{
$this->searchSelection = $doctor;
$this->selectionType = 'doctor';
$this->showDocSpeList = false;
}
public function render()
{
return view('livewire.resources-search');
}
}
Summary of the Code Implementation
This section outlines the steps to implement a multi table searchable dropdown using Livewire. Here’s a concise breakdown of the process:
- Create Livewire Component: Generate a Livewire component to handle the search functionality. This component will manage user input and data retrieval from the database.
- Define Properties: In the Livewire class, define properties for storing search results, user input, and visibility of the dropdown list. This includes arrays for matching doctors, specializations, and exams, as well as flags for controlling UI behavior.
- Implement Search Logic: Create a method (
searchDocsAndSpecializations
) that triggers on user input. This method queries the database for matching doctors, specializations, and exams based on the user’s search term. Reset the results if the input is empty to keep the UI clean. - Handle Selection: Implement methods (
selectDoctor
,selectSpecialization
,selectExam
) to handle user selections from the dropdown. These methods update the main selection and hide the dropdown. - Create the View: In the Livewire view, set up an input field for user search, and display the results in a dropdown list. Use conditional rendering to manage the visibility of the list based on the search results.
- Style the Dropdown: Ensure the dropdown is styled appropriately to match your application’s design, providing a seamless user experience. We used bootstrap for this project.
By following these steps, you can effectively integrate a multi table searchable dropdown into your application, enhancing user accessibility and interaction.
Get in Touch
If you’re looking to enhance your mobile or web application’s UI/UX design in Figma, or if you need expert assistance in improving your existing designs, feel free to reach out to us at info@theuistudio.com. We specialize in creating user-centered designs that elevate user experiences. If you are planning to get a pixel-perfect HTML/CSS/Bootstrap website for your Figma design, we can help you implement robust solutions tailored to your needs. Let’s collaborate to bring your vision to life!
FAQs
What are the Key Benefits of Using a Multi table Searchable Dropdown in a Healthcare Application?
The multi-table searchable dropdown streamlines user experience by allowing quick access to various categories like doctors, specializations, and exams in one interface. It reduces cognitive load, minimizes navigation complexity, and enhances the efficiency of information retrieval. This is particularly crucial in healthcare settings, where users often need to find information rapidly.
How does this Feature Improve User Engagement Compared to Traditional Dropdowns?
By providing real-time suggestions and displaying relevant results as users type, the multi-table dropdown encourages interaction and keeps users engaged. Traditional dropdowns, which may require extensive scrolling or switching between categories, can lead to frustration and disengagement, whereas our implementation fosters a more intuitive and satisfying search experience.
What Considerations were Made to Ensure Accessibility in the Design?
The dropdown is designed to be responsive and accessible (using Bootstrap), ensuring that all users can easily navigate and interact with it. Features like keyboard navigation support, clear labeling, and immediate feedback on input enhance usability for individuals with varying abilities, aligning with best practices for inclusive design.
How Does the Implementation of this Feature Impact the Overall Maintenance of the Application?
While the multi-table searchable dropdown does involve multiple queries, it simplifies the user interface, reducing the need for extensive separate components. This unified approach makes it easier to maintain and update the codebase, allowing developers to focus on enhancing functionality rather than managing fragmented elements across the application.