Tired of boring, conventional dropdowns? Are your clients asking for live search or searchable dropdowns instead 🔍?
We recently tackled this challenge for a client and thought it was worth sharing (developing a searchable dropdown with Livewire). At The UI Studio, one of our core goals is to enhance user experience in every way possible. This means going beyond visually appealing front-end designs and treating UX with the same importance as UI. Sometimes, that even requires optimizing back-end logic to deliver a seamless experience. In this blog, we’ll walk you through a real-world use case, the limitations of the old approach, and the new solution our client loved 😍. If a Figma expert has provided you with a searchable dropdown UI and you’re a Laravel/Livewire developer looking to upgrade standard dropdowns into user-friendly, searchable lists, this guide is for you!
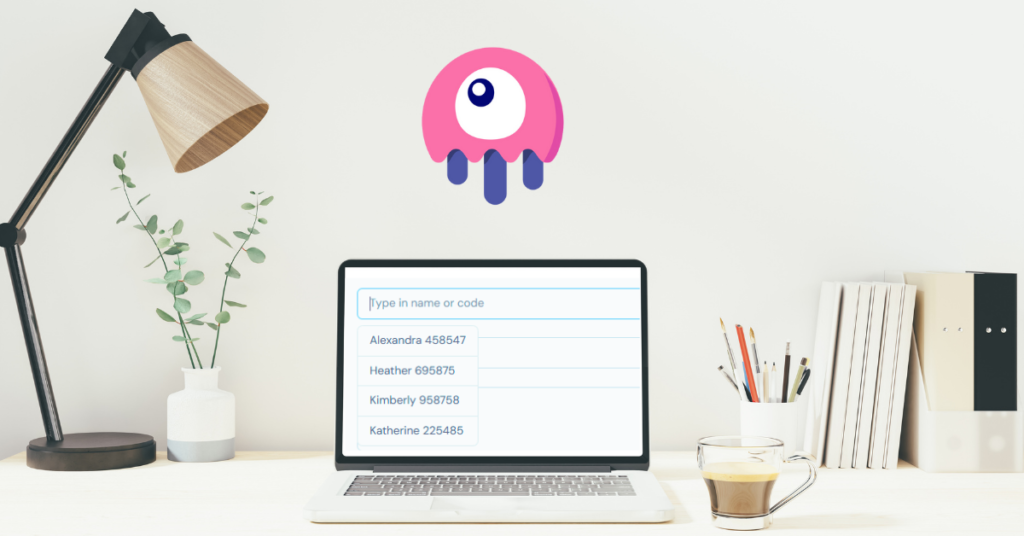
Elevating UX by Searchable Dropdown with Livewire
Searchable dropdowns significantly improve the user experience. Imagine you need to select a landlord from a long list scrolling endlessly up and down just to find the one you need. Feels frustrating, right? 😫 Let’s face it: it’s a hassle!
Understanding the Use-Case Where We Needed a Searchable Dropdown
Our developers were working on a Tenant Management System where the “Properties” module required linking each property to its corresponding landlord. Admins would need to select a landlord from the “Landlord” module, where each landlord had a unique code and details.
Old Approach for the Dropdown
Take a look at the image below. This was the old dropdown approach. Our UI/UX team wasn’t a fan either, but we only planned to change it after the client gave us the green light. The client was happy with the old approach, but we were already anticipating limitations as the list of landlords continued to grow. 🤔

Old Backend and UI Code for the Dropdown in Livewire
Let’s take a look at the old code behind the conventional dropdown.
In Livewire class:
// Define a public property to hold the list of landlords
public $landlords;
// Method to retrieve landlords
public function landlords()
{
// Check if the landlords property is empty
if (empty($this->landlords))
{
// fetch all landlords from the database and assign to the property
$this->landlords = Landlord::all();
}
// The landlords property will now contain all landlords from the database
}
On client side:
<div class="mb-4 row align-items-center">
<label class="form-label col-sm-3 col-form-label">Select Landlord</label>
<div class="col-sm-9">
<select wire:model.defer="landlord_id" class="form-control">
<option value="">Select</option>
@foreach($landlords as $landlord)
<option value="{{ $landlord->id }}">
{{ $landlord->full_name }} ({{ $landlord->code}})
</option>
@endforeach
</select>
</div>
</div>
Leveraging Livewire to Transform Conventional Dropdowns into Searchable Dropdown/Lists
After receiving the client’s request to transform the dropdown into a searchable dropdown or list, we were excited to dive into the solution. Since the project was built using Laravel, Livewire, MySQL for the backend, and Bootstrap/HTML/CSS for the front end, our Livewire experts quickly devised a plan. The solution didn’t just allow searching landlords by name; it also enabled filtering by their unique IDs. Looking for a way to implement multi-table searchable dropdown lists in Livewire? Take a look at this multi-table searchable dropdown article.
Searchable Dropdown with Livewire: Features
- Search using Landlord names
- Search using Landlord IDs/code
- Show all Landlords when click in the searchable field by default
Show Landlords by Default
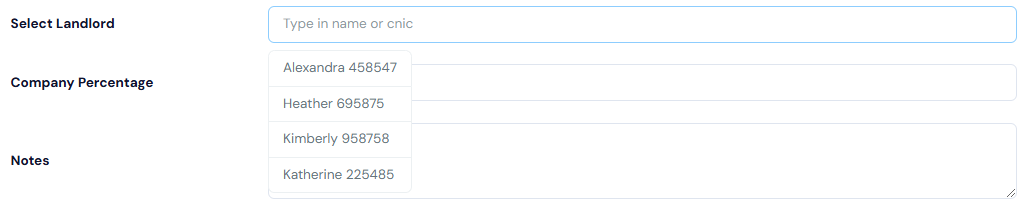
Search with Landlords Names


Search with Landlords Codes


UI and Backend Code Enhancements for the Searchable Dropdown in Livewire
Let’s review the code enhancement that our team did.
In Livewire class:
public function searchLandlord()
{
if ($this->landlord_name) {
$this->matchingLandlords = Landlord::select('id', 'full_name', 'code')
->where('full_name', 'like', '%' . $this->landlord_info . '%')
->orWhere('code', 'like', '%' . $this->landlord_info . '%')
->get();
} else {
$this->matchingLandlords = []; // Clear previous results if no input
}
}
public function showAllLandlords()
{
$this->matchingLandlords = Landlord::select('id', 'full_name', 'code')
->take(10)
->get();
}
On client side:
<div class="mb-4 row align-items-center">
<label class="form-label col-sm-3 col-form-label">Select Landlord</label>
<div class="col-sm-9">
<input type="text"
wire:model.defer="landlord_info"
wire:keyup="searchLandlord"
wire:focus="showAllLandlords"
placeholder="Type in name or code"
class="form-control"
>
<ul class="list-group mt-2 position-absolute" style="display: {{ count($matchingLandlords) > 0 ? 'block' : 'none' }}; z-index: 1000; max-width: 300px; max-height: 200px; overflow-y: auto;">
@if(count($matchingLandlords) > 0)
@foreach($matchingLandlords as $landlord)
<li class="list-group-item" wire:click="selectLandlord('{{ $landlord['id'] }}')" style="cursor: pointer;">
{{ $landlord['full_name'] }} {{$landlord['code']}}
</li>
@endforeach
@else
<li class="list-group-item">No landlord found</li>
@endif
</ul>
</div>
</div>
Livewire Searchable Dropdown Code Explanation
The above Laravel/Livewire code consists of two methods within a class, for managing landlord data.
Method Descriptions:
searchLandlord()
:- Purpose: Searches for landlords based on user input.
- Functionality:
- If the
landlord_name
property is set (not empty), it queries theLandlord
model for records where thefull_name
orcnic
contains the input text. - The results are stored in the
matchingLandlords
property. - If there is no input, it clears the
matchingLandlords
array.
- If the
showAllLandlords()
:- Purpose: Retrieves a default list of landlords.
- Functionality:
- It fetches the first 10 landlord records from the database and assigns them to the
matchingLandlords
property.
- It fetches the first 10 landlord records from the database and assigns them to the
Explanation of the Livewire Blade/View Component
- Input Field:
- The input field is bound to the
landlord_info
property usingwire:model.defer
. This means that changes to the input will be sent to the Livewire component only when the input loses focus. - The
wire:keyup
directive triggers thesearchLandlord
method as the user types, allowing for live searching. - The
wire:focus
directive calls theshowAllLandlords
method when the input is focused, which populates the dropdown with a default list of landlords.
- The input field is bound to the
- Dropdown List:
- An unordered list (
<ul>
) displays the search results. It only shows when there are matching landlords(count($matchingLandlords) > 0)
. - Each landlord is listed as a clickable item (
<li>
). When a user clicks on a landlord, it calls theselectLandlord
method, passing the landlord’s ID.
- An unordered list (
Code Summary
Together, the Livewire class and the view code create a dynamic and responsive interface for selecting landlords. The Livewire methods manage the data fetching and state management, while the view handles user interaction and display. This setup allows users to search for landlords efficiently and select them from a live-updated list.
Ready to Elevate Your UI/UX?
If you’re looking to enhance user experience with sleek, intuitive designs in Figma and pixel-perfect front-end development, The UI Studio is here to help! We specialize in crafting engaging and efficient experiences. Contact us today at info@theuistudio.com and let’s bring your ideas to life! 🚀
Explore Our UI/UX Work and Connect with Us
We believe in sharing our design journey and showcasing our projects across various platforms. You can explore our latest work, get inspired, or connect with us through the following links:
- Behance: Browse our portfolio for in-depth project showcases.
- Instagram: Stay updated with our latest designs and creative processes.
- LinkedIn: Connect with us professionally and discover our industry expertise.
- Website: Visit our website for more about our services and offerings.
Feel free to reach out and engage with us on any platform, or comment below here on the blog! We’d love to hear from you!